Blog -
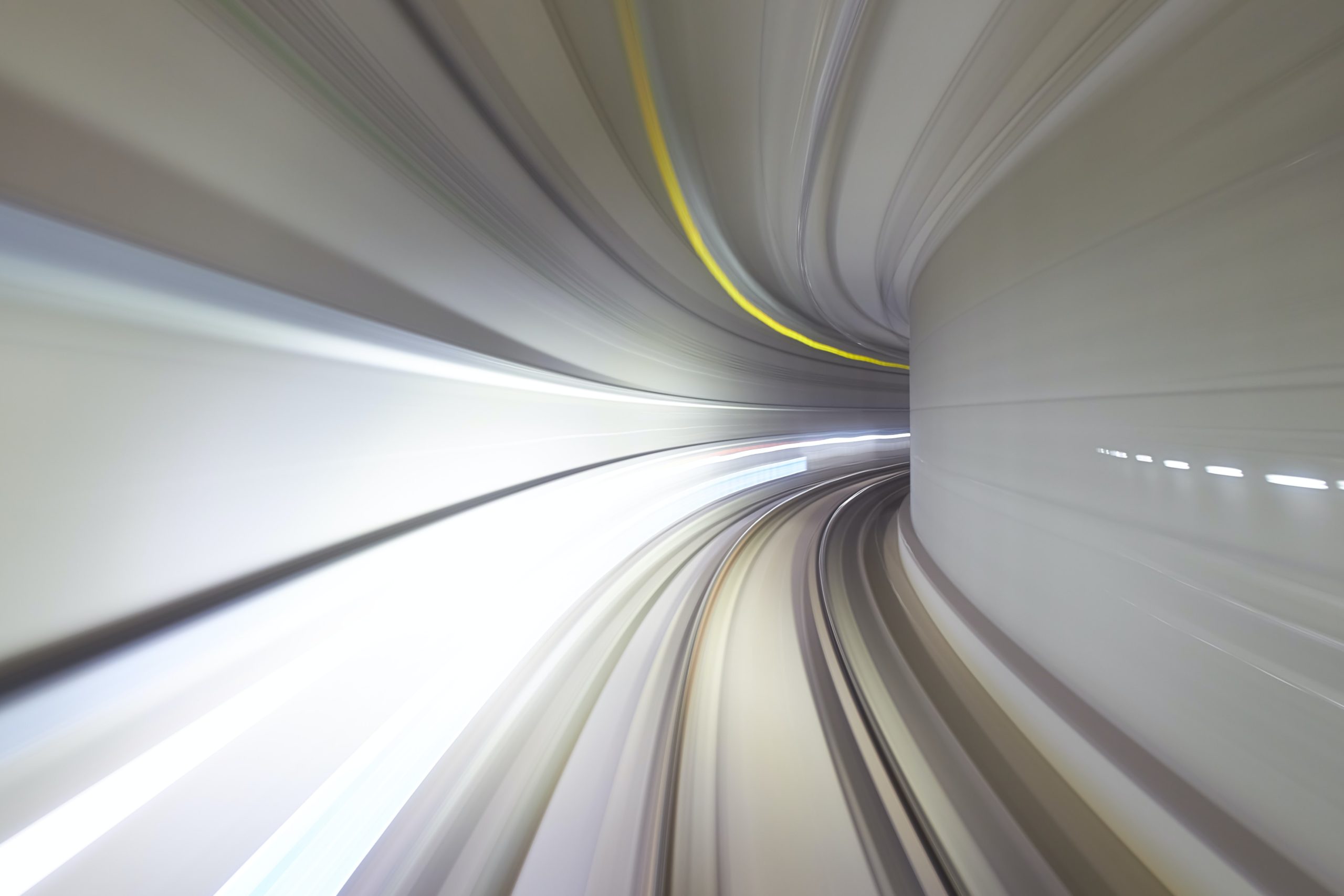
Migrating the Google Drive API from v2 to v3
When integrating an application with external data sources, developers need to be conscious of how an API request may impact performance. If you are not careful, you could easily receive responses that contain lots of unnecessary data, resulting in payload sizes that increase memory costs and network latency rates.
Here at FloQast, we’ve been going through the process of transitioning large portions of our codebase to smaller repositories on both the backend and the frontend. Since some of our code was no longer stored in a back-end monolith, we could notice a delay in some of our data requests. As a result, we were presented with the challenge of streamlining our integrations as much as possible in order to provide the same data our processes needed, without causing a lag for our customers.
For these reasons, we recently migrated from version 2 to version 3 of the Google Drive API. In this article, we’ll discuss how v3 optimizes performance and the small changes needed to transition an app from v2 to v3.
Why Should You Upgrade to v3?
One of the primary differences between v2 and v3 is that v3 improves performance by returning a limited amount of data by default. This is a big change from v2, where searching for files would return all file metadata by default. To further encourage this optimization, most methods that return a response now require that you specify the fields you need. Receiving only relevant data in this fashion makes life easier for developers, since they will no longer have to weed through a massive JSON response, and it also allows the API to function more efficiently, which delivers responses faster.
Let’s See This Optimization In Action
Say we wanted to search for all files in a Google Drive folder in order for our app to create links to the files with icons matching their file types. We want to create a method that returns an array of folder data objects containing the file id for the link, the file name to label the link, and the mimeType to determine what icon to assign.
Here’s how we can accomplish that using Google Drive API v2:
/** * Lists all fields of files * @param {google.auth.OAuth2} auth An authorized OAuth2 client. */ async function listFilesV2(auth) { const drive = google.drive({version: 'v2', auth}); try { const res = await drive.files.list(); const files = res.data.items; let fileData = []; if (files.length) { files.map((file) => { fileData.push({ id: file.id, name: file.title, mimeType: file.mimeType }); }); } return fileData; } catch (err) { return console.log('The API returned an error: ' + err); } };
This gives us the data we are looking for. However, by default, our v2 call would also return more than 30 other data fields that we don’t need…for each file!
With a few slight changes to our request, we can use v3 to get a much more streamlined response:
/** * Lists kind, id, name, mimetype of files * @param {google.auth.OAuth2} auth An authorized OAuth2 client. */ async function listFilesV3(auth) { const drive = google.drive({version: 'v3', auth}); try { const res = await drive.files.list(); const files = res.data.files; let fileData = []; if (files.length) { files.map((file) => { fileData.push({ id: file.id, name: file.name, mimeType: file.mimeType }); }); } return fileData; } catch (err) { return console.log('The API returned an error: ' + err); } };
Even without specifying the fields we want, v3 returns only 4 fields (kind, id, name, mimeType) by default. Unlike the result from v2, we aren’t left with a huge amount of unused data that slowed down our response. We can even further optimize this by using the fields parameter on our request. For example, say we change our use case and only need the file id and name. Here’s how we can trim down our response to only request those fields:
/** * Lists id & name of files * @param {google.auth.OAuth2} auth An authorized OAuth2 client. */ async function listFilesV3Filtered(auth) { const drive = google.drive({version: 'v3', auth}); try { const res = await drive.files.list({ fields: 'files(id, name)' }); const files = res.data.files; let fileData = []; if (files.length) { files.map((file) => { fileData.push({ id: file.id, name: file.name, mimeType: file.mimeType }); }); } return fileData; } catch (err) { return console.log('The API returned an error: ' + err); } };
How to Migrate from v2 to v3
As you can see, the transition from v2 to v3 wasn’t a lot of work, but it optimized our request by removing approximately 30 data fields per file by default. That’s a huge performance optimization that will definitely help our customers as our application grows and needs to handle more data. Here are the steps we took to migrate our v2 method to v3:
- Changed our google.drive version string from v2 to v3
- Retrieved the file data from our v3 response’s data.files instead of v2’s data.items
- Set the name property from our v3 response’s file.name instead of v2’s file.title
That’s it! Pretty simple right? Google also made a number of changes to v3 to improve the API’s naming conventions and to remove duplicate functionality. For example:
- The files.list method provides the same functionality that v2’s Children and Parents collections did, so those collections have been removed
- The Properties collection has also been removed since the Files resource already has the properties field providing the same data
- To create a file, instead of using v2’s files.insert method, we now use v3’s files.create
- For more v2 to v3 naming changes, view this reference guide
With more intuitive naming, removed redundancies, and streamlined responses, v3 provides lots of benefits to developers and is definitely worth the small effort to migrate.
Back to Blog